C++ の if、if...else およびネストされた if...else
C++ の if, if...else とネストされた if...else
このチュートリアルでは、例を使用して意思決定プログラムを作成する if...else ステートメントについて学習します。
コンピュータ プログラミングでは、if...else
を使用します 特定の条件下で 1 つのコード ブロックを実行し、別の条件下で別のコード ブロックを実行するステートメント。
たとえば、学生が取得した点数に基づいて成績 (A、B、C) を割り当てます。
- パーセンテージが 90 を超える場合 、評価 A を割り当てます
- パーセンテージが 75 を超える場合 、評価 B を割り当てます
- パーセンテージが 65 を超える場合 、グレード C を割り当てます
if...else
には 3 つの形式があります C++ のステートメント。
if
声明if...else
声明if...else if...else
声明C++ if ステートメント
if
の構文 ステートメントは:
if (condition) {
// body of if statement
}
if
ステートメントは condition
を評価します 括弧内 ( )
.
condition
の場合true
に評価されます 、if
の本体内のコード 実行されます。condition
の場合false
に評価されます 、if
の本体内のコード スキップされます。
注: { }
内のコード if
の本体です
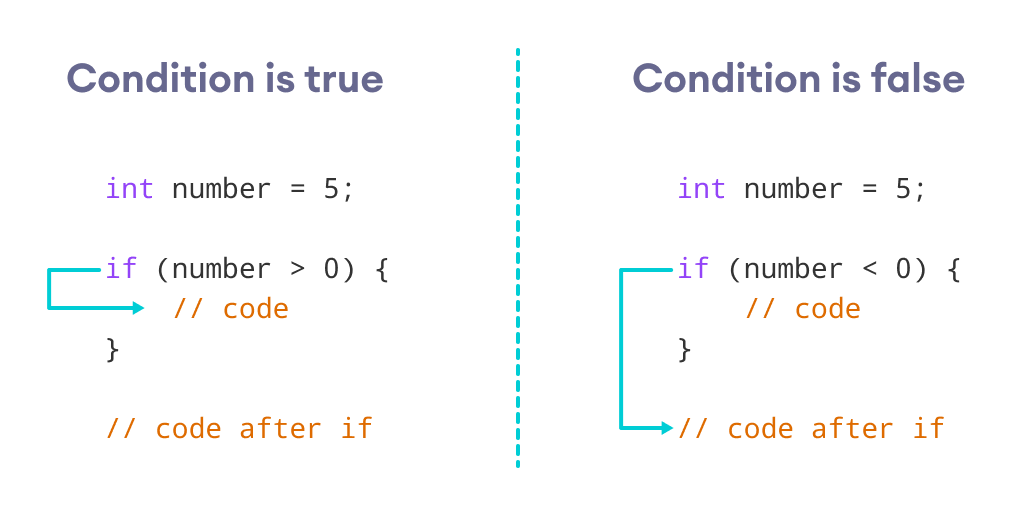
例 1:C++ の if ステートメント
// Program to print positive number entered by the user
// If the user enters a negative number, it is skipped
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter an integer: ";
cin >> number;
// checks if the number is positive
if (number > 0) {
cout << "You entered a positive integer: " << number << endl;
}
cout << "This statement is always executed.";
return 0;
}
出力 1
Enter an integer: 5 You entered a positive number: 5 This statement is always executed.
ユーザーが 5
を入力したとき 、条件 number > 0
true
に評価されます if
の本体内のステートメント 実行されます。
アウトプット 2
Enter a number: -5 This statement is always executed.
ユーザーが -5
を入力したとき 、条件 number > 0
false
に評価されます および if
の本文内のステートメント 実行されません。
C++ if...else
if
ステートメントにはオプションの else
を含めることができます 句。その構文は次のとおりです:
if (condition) {
// block of code if condition is true
}
else {
// block of code if condition is false
}
if..else
ステートメントは condition
を評価します
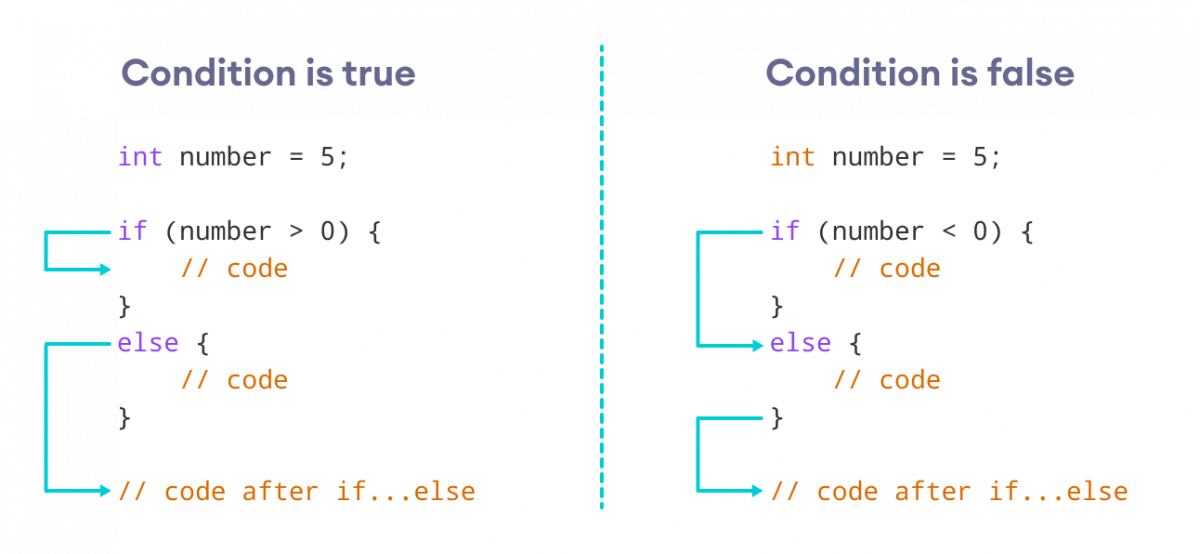
condition
の場合 true
を評価します 、
if
の本体内のコード 実行されますelse
の本体内のコード 実行からスキップされます
condition
の場合 false
を評価します 、
else
の本体内のコード 実行されますif
の本体内のコード 実行からスキップされます
例 2:C++ の if...else ステートメント
// Program to check whether an integer is positive or negative
// This program considers 0 as a positive number
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter an integer: ";
cin >> number;
if (number >= 0) {
cout << "You entered a positive integer: " << number << endl;
}
else {
cout << "You entered a negative integer: " << number << endl;
}
cout << "This line is always printed.";
return 0;
}
出力 1
Enter an integer: 4 You entered a positive integer: 4. This line is always printed.
上記のプログラムでは、条件 number >= 0
があります。 . 0以上の数値を入力すると の場合、条件は true
と評価されます .
ここでは、4 と入力します .したがって、条件は true
です .したがって、 if
の本体内のステートメント 実行されます。
アウトプット 2
Enter an integer: -4 You entered a negative integer: -4. This line is always printed.
ここでは、-4 と入力します。 .したがって、条件は false
です .したがって、 else
の本体内のステートメント 実行されます。
C++ の if...else...else if ステートメント
if...else
ステートメントは、2 つの選択肢の間でコードのブロックを実行するために使用されます。ただし、2 つ以上の選択肢から選択する必要がある場合は、if...else if...else
を使用します。
if...else if...else
の構文 ステートメントは:
if (condition1) {
// code block 1
}
else if (condition2){
// code block 2
}
else {
// code block 3
}
ここで、
- If
condition1
true
に評価されます 、code block 1
実行されます。 - If
condition1
false
に評価されます 、次にcondition2
評価されます。 - If
condition2
true
です 、code block 2
実行されます。 - If
condition2
false
です 、code block 3
実行されます。
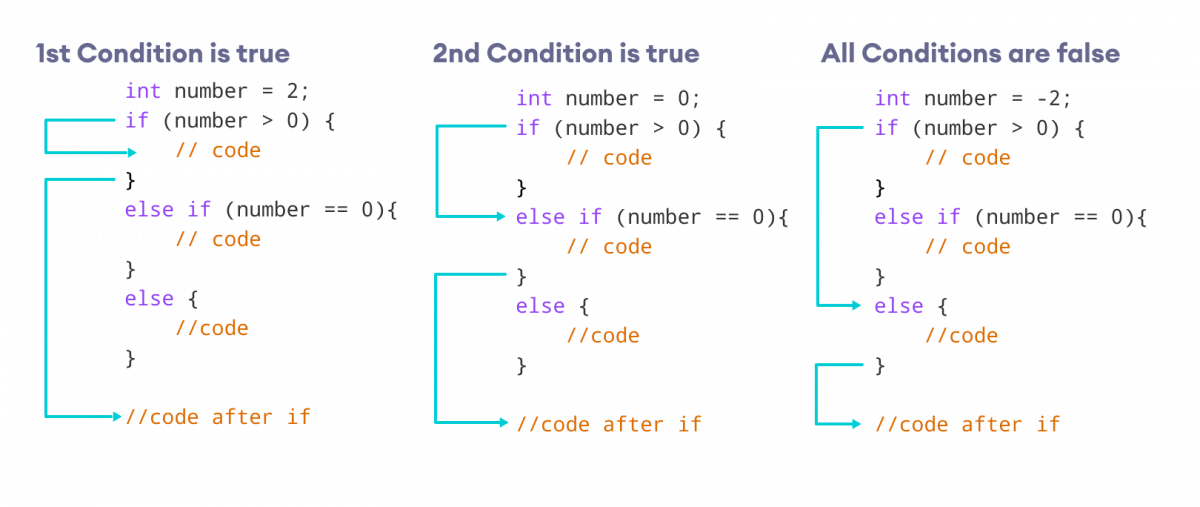
注: 複数の else if
が存在する可能性があります ステートメントですが、if
は 1 つだけです そして else
例 3:C++ の if...else...else if
// Program to check whether an integer is positive, negative or zero
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter an integer: ";
cin >> number;
if (number > 0) {
cout << "You entered a positive integer: " << number << endl;
}
else if (number < 0) {
cout << "You entered a negative integer: " << number << endl;
}
else {
cout << "You entered 0." << endl;
}
cout << "This line is always printed.";
return 0;
}
出力 1
Enter an integer: 1 You entered a positive integer: 1. This line is always printed.
アウトプット 2
Enter an integer: -2 You entered a negative integer: -2. This line is always printed.
アウトプット 3
Enter an integer: 0 You entered 0. This line is always printed.
このプログラムでは、ユーザーから番号を取得します。次に if...else if...else
を使用します はしごを使って、数値が正か負かゼロかを確認します。
数値が 0
より大きい場合 、 if
内のコード ブロックが実行されます。数値が 0
未満の場合 、 else if
内のコード ブロックが実行されます。それ以外の場合、else
内のコード ブロックが実行されます。
C++ ネストされた if...else
if
を使用する必要がある場合もあります 別の if
内のステートメント 声明。これは、ネストされた if
として知られています。
if
の複数のレイヤーと考えてください ステートメント。最初の外側の if
があります ステートメントであり、その内部には別の内部 if
があります 声明。その構文は次のとおりです:
// outer if statement
if (condition1) {
// statements
// inner if statement
if (condition2) {
// statements
}
}
注:
else
を追加できます とelse if
内部if
へのステートメント 必要に応じて声明- 内側の
if
ステートメントは外側のelse
の中に挿入することもできます またはelse if
ステートメント (存在する場合) if
の複数のレイヤーをネストできます
例 4:C++ のネストされた if
// C++ program to find if an integer is positive, negative or zero
// using nested if statements
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter an integer: ";
cin >> num;
// outer if condition
if (num != 0) {
// inner if condition
if (num > 0) {
cout << "The number is positive." << endl;
}
// inner else condition
else {
cout << "The number is negative." << endl;
}
}
// outer else condition
else {
cout << "The number is 0 and it is neither positive nor negative." << endl;
}
cout << "This line is always printed." << endl;
return 0;
}
出力 1
Enter an integer: 35 The number is positive. This line is always printed.
アウトプット 2
Enter an integer: -35 The number is negative. This line is always printed.
アウトプット 3
Enter an integer: 0 The number is 0 and it is neither positive nor negative. This line is always printed.
上記の例では、
- ユーザーからの入力として整数を受け取り、それを変数 num に格納します .
- 次に
if...else
を使用します num が0
と等しくないかどうかをチェックするステートメント .- If
true
、次に内側if...else
ステートメントが実行されます。 - If
false
、outer 内のコードelse
条件が実行され、"The number is 0 and it is neither positive nor negative."
が出力されます
- If
- インナー
if...else
ステートメントは、入力数値が正かどうか、つまり num かどうかをチェックします 0 より大きい .- If
true
の場合、数値が正であることを示すステートメントを出力します。 - If
false
、数値が負であることを出力します。
- If
注: ご覧のとおり、ネストされた if...else
ロジックを複雑にします。可能であれば、ネストされた if...else
を常に避けるようにしてください。 .
ステートメントが 1 つだけの if...else の本体
if...else
の本体の場合 { }
は省略できます。 プログラムで。たとえば、
int number = 5;
if (number > 0) {
cout << "The number is positive." << endl;
}
else {
cout << "The number is negative." << endl;
}
と
int number = 5;
if (number > 0)
cout << "The number is positive." << endl;
else
cout << "The number is negative." << endl;
両方のプログラムの出力は同じになります。
注: { }
を使用する必要はありませんが if...else
の本体の場合 { }
を使用して、ステートメントが 1 つだけあります コードが読みやすくなります。
意思決定の詳細
特定の状況では、三項演算子 if...else
を置き換えることができます 声明。詳細については、C++ 三項演算子をご覧ください。
与えられたテスト条件に基づいて複数の選択肢から選択する必要がある場合、switch
ステートメントを使用できます。詳細については、C++ スイッチにアクセスしてください。
詳細については、次の例をご覧ください:
数値が偶数か奇数かをチェックする C++ プログラム
文字が母音か子音かをチェックする C++ プログラム。
3 つの数の中から最大の数を見つける C++ プログラム
C言語