C++ 関数のオーバーライド
C++ 関数のオーバーライド
このチュートリアルでは、例を使用して C++ での関数のオーバーライドについて学習します。
ご存じのとおり、継承は基本クラスから派生クラスを作成できるようにする OOP の機能です。派生クラスは基本クラスの機能を継承します。
派生クラスと基底クラスの両方で同じ関数が定義されているとします。ここで、派生クラスのオブジェクトを使用してこの関数を呼び出すと、派生クラスの関数が実行されます。
これは、関数のオーバーライドとして知られています C++で。派生クラスの関数は、基本クラスの関数をオーバーライドします。
例 1:C++ 関数のオーバーライド
// C++ program to demonstrate function overriding
#include <iostream>
using namespace std;
class Base {
public:
void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
}
};
int main() {
Derived derived1;
derived1.print();
return 0;
}
出力
Derived Function
ここで、同じ関数 print()
Base
の両方で定義されています と Derived
クラス。
print()
を呼び出すと、 Derived
から オブジェクト派生1 、print()
Derived
から Base
の関数をオーバーライドして実行されます .
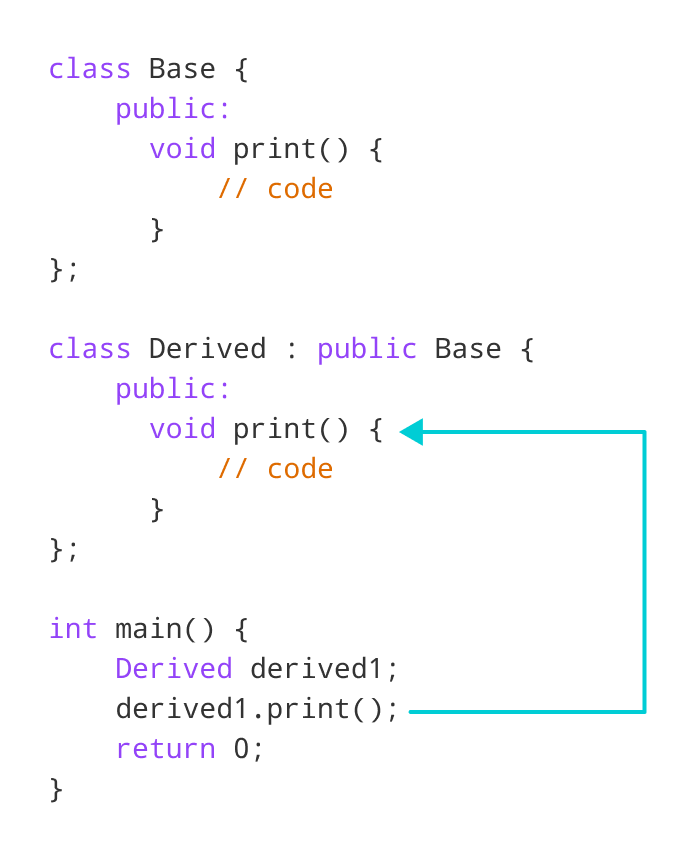
ご覧のとおり、Derived
のオブジェクトから関数を呼び出したため、関数はオーバーライドされました。 クラス。
print()
を呼び出していたら Base
のオブジェクトからの関数 クラスでは、関数はオーバーライドされませんでした。
// Call function of Base class
Base base1;
base1.print(); // Output: Base Function
C++ でオーバーライドされた関数にアクセスする
基本クラスのオーバーライドされた関数にアクセスするには、スコープ解決演算子 ::
を使用します .
基本クラスのポインターを使用して派生クラスのオブジェクトをポイントし、そのポインターから関数を呼び出すことによって、オーバーライドされた関数にアクセスすることもできます。
例 2:基本クラスへのオーバーライドされた関数への C++ アクセス
// C++ program to access overridden function
// in main() using the scope resolution operator ::
#include <iostream>
using namespace std;
class Base {
public:
void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
}
};
int main() {
Derived derived1, derived2;
derived1.print();
// access print() function of the Base class
derived2.Base::print();
return 0;
}
出力
Derived Function Base Function
ここで、このステートメント
derived2.Base::print();
print()
にアクセスします 基本クラスの機能。
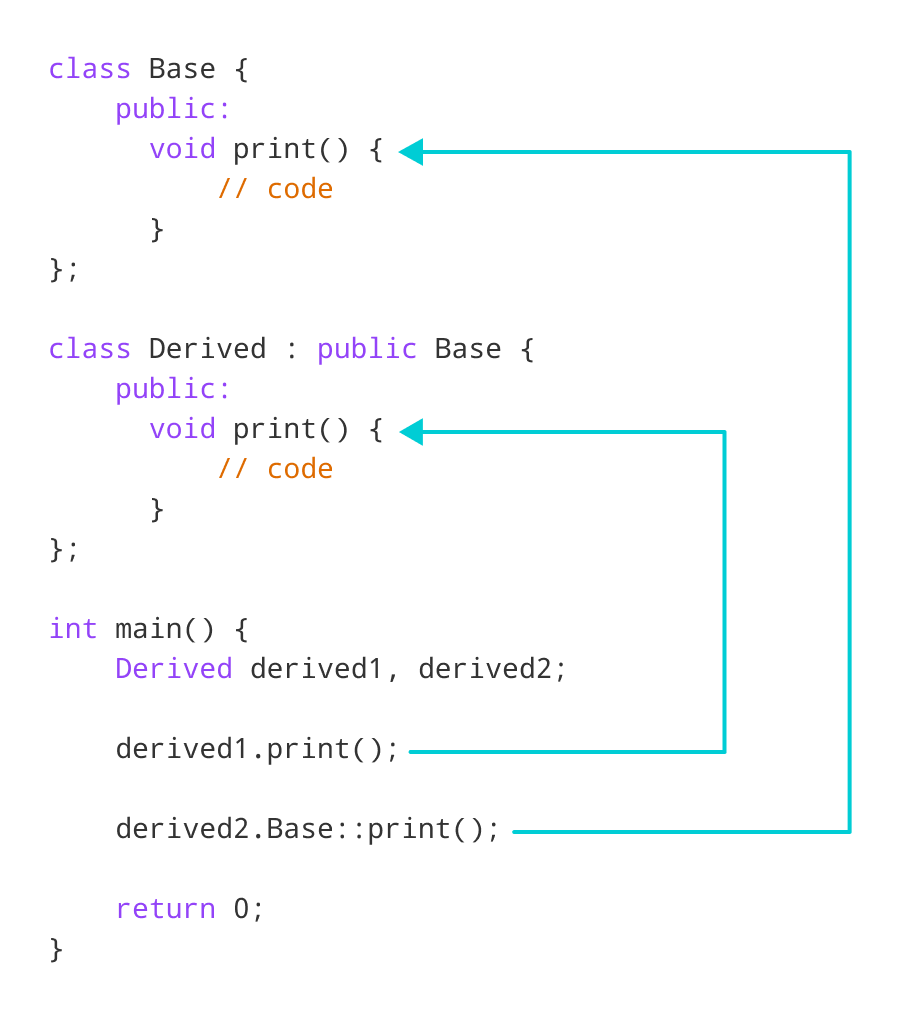
例 3:派生クラスからオーバーライドされた関数を C++ で呼び出す
// C++ program to call the overridden function
// from a member function of the derived class
#include <iostream>
using namespace std;
class Base {
public:
void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
// call overridden function
Base::print();
}
};
int main() {
Derived derived1;
derived1.print();
return 0;
}
出力
Derived Function Base Function
このプログラムでは、オーバーライドされた関数を Derived
内で呼び出しています。 クラス自体。
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
Base::print();
}
};
コード Base::print();
に注意してください Derived
内のオーバーライドされた関数を呼び出します クラス。
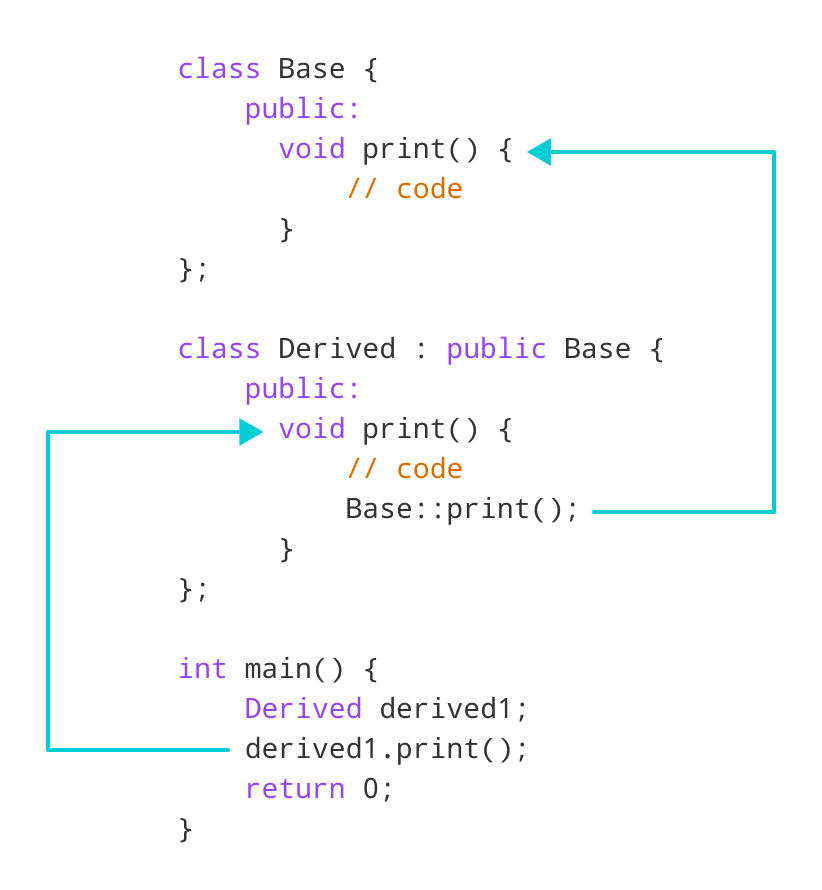
例 4:ポインターを使用してオーバーライドされた関数を C++ で呼び出す
// C++ program to access overridden function using pointer
// of Base type that points to an object of Derived class
#include <iostream>
using namespace std;
class Base {
public:
void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
}
};
int main() {
Derived derived1;
// pointer of Base type that points to derived1
Base* ptr = &derived1;
// call function of Base class using ptr
ptr->print();
return 0;
}
出力
Base Function
このプログラムでは、Base
のポインターを作成しました。 ptr という名前の型 .このポインタは Derived
を指しています オブジェクト派生1 .
// pointer of Base type that points to derived1
Base* ptr = &derived1;
print()
を呼び出すと、 ptr を使用した関数 、オーバーライドされた関数を Base
から呼び出します .
// call function of Base class using ptr
ptr->print();
これは ptr Derived
を指す オブジェクト、実際には Base
のものです タイプ。 Base
のメンバー関数を呼び出します。 .
Base
をオーバーライドするには 関数にアクセスする代わりに、Base
で仮想関数を使用する必要があります。 クラス。
C言語