Java-継承
継承は、あるクラスが別のクラスのプロパティ (メソッドとフィールド) を取得するプロセスとして定義できます。継承を使用することで、情報は階層的な順序で管理しやすくなります。
他のプロパティを継承するクラスはサブクラス (派生クラス、子クラス) と呼ばれ、プロパティが継承されるクラスはスーパークラス (基底クラス、親クラス) と呼ばれます。
拡張キーワード
延長 クラスのプロパティを継承するために使用されるキーワードです。以下は extends キーワードの構文です。
構文
class Super { ..... ..... } class Sub extends Super { ..... ..... }
サンプル コード
以下は、Java 継承を示す例です。この例では、Calculation と My_Calculation という 2 つのクラスを確認できます。
extends キーワードを使用して、My_Calculation は Calculation クラスのメソッド add() および Subtraction() を継承します。
次のプログラムをコピーして、My_Calculation.java という名前のファイルに貼り付けます
例
ライブデモclass Calculation { int z; public void addition(int x, int y) { z = x + y; System.out.println("The sum of the given numbers:"+z); } public void Subtraction(int x, int y) { z = x - y; System.out.println("The difference between the given numbers:"+z); } } public class My_Calculation extends Calculation { public void multiplication(int x, int y) { z = x * y; System.out.println("The product of the given numbers:"+z); } public static void main(String args[]) { int a = 20, b = 10; My_Calculation demo = new My_Calculation(); demo.addition(a, b); demo.Subtraction(a, b); demo.multiplication(a, b); } }
以下に示すように、上記のコードをコンパイルして実行します。
javac My_Calculation.java java My_Calculation
プログラムを実行すると、次の結果が生成されます-
出力
The sum of the given numbers:30 The difference between the given numbers:10 The product of the given numbers:200
与えられたプログラムで、My_Calculation へのオブジェクトが クラスが作成されると、その中にスーパークラスの内容のコピーが作成されます。そのため、サブクラスのオブジェクトを使用してスーパークラスのメンバーにアクセスできます。
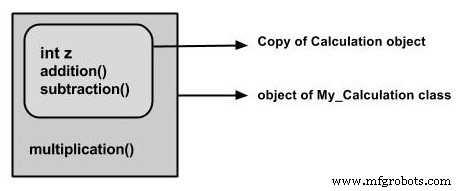
スーパークラス参照変数はサブクラス オブジェクトを保持できますが、その変数を使用してアクセスできるのはスーパークラスのメンバーのみです。そのため、両方のクラスのメンバーにアクセスするには、常にサブクラスへの参照変数を作成することをお勧めします。
上記のプログラムを考えると、以下のようにクラスをインスタンス化できます。ただし、スーパークラス参照変数 ( cal この場合) メソッド multiplication() を呼び出すことはできません サブクラス My_Calculation に属します。
Calculation demo = new My_Calculation(); demo.addition(a, b); demo.Subtraction(a, b);
注意 − サブクラスは、そのスーパークラスからすべてのメンバー (フィールド、メソッド、およびネストされたクラス) を継承します。コンストラクターはメンバーではないため、サブクラスに継承されませんが、スーパークラスのコンストラクターはサブクラスから呼び出すことができます。
スーパー キーワード
スーパー キーワードはこれに似ています キーワード。以下は、super キーワードが使用されるシナリオです。
- <リ>
メンバーを区別するために使用されます
<リ>スーパークラスの呼び出しに使用されます サブクラスからのコンストラクター。
メンバーの差別化
クラスが別のクラスのプロパティを継承している場合。スーパークラスのメンバーがサブクラスと同じ名前を持つ場合、これらの変数を区別するために、以下に示すようにスーパー キーワードを使用します。
super.variable super.method();
サンプル コード
このセクションでは、super の使用方法を示すプログラムを提供します。 キーワード。
このプログラムには、Sub_class という 2 つのクラスがあります。 そしてスーパークラス どちらも、異なる実装を持つ display() という名前のメソッドと、異なる値を持つ num という名前の変数を持っています。両方のクラスの display() メソッドを呼び出し、両方のクラスの変数 num の値を出力しています。ここで、スーパークラスのメンバーとサブクラスを区別するために super キーワードを使用していることがわかります。
プログラムをコピーして、Sub_class.java という名前のファイルに貼り付けます。
例
ライブデモclass Super_class { int num = 20; // display method of superclass public void display() { System.out.println("This is the display method of superclass"); } } public class Sub_class extends Super_class { int num = 10; // display method of sub class public void display() { System.out.println("This is the display method of subclass"); } public void my_method() { // Instantiating subclass Sub_class sub = new Sub_class(); // Invoking the display() method of sub class sub.display(); // Invoking the display() method of superclass super.display(); // printing the value of variable num of subclass System.out.println("value of the variable named num in sub class:"+ sub.num); // printing the value of variable num of superclass System.out.println("value of the variable named num in super class:"+ super.num); } public static void main(String args[]) { Sub_class obj = new Sub_class(); obj.my_method(); } }
次の構文を使用して、上記のコードをコンパイルして実行します。
javac Super_Demo java Super
プログラムを実行すると、次の結果が得られます-
出力
This is the display method of subclass This is the display method of superclass value of the variable named num in sub class:10 value of the variable named num in super class:20
スーパークラス コンストラクターの呼び出し
クラスが別のクラスのプロパティを継承している場合、サブクラスは自動的にスーパークラスのデフォルト コンストラクターを取得します。ただし、スーパークラスのパラメーター化されたコンストラクターを呼び出したい場合は、以下に示すように super キーワードを使用する必要があります。
super(values);
サンプル コード
このセクションで示すプログラムは、スーパー キーワードを使用して、スーパークラスのパラメーター化されたコンストラクターを呼び出す方法を示しています。このプログラムにはスーパークラスとサブクラスが含まれており、スーパークラスには整数値を受け入れるパラメーター化されたコンストラクターが含まれており、スーパー キーワードを使用してスーパークラスのパラメーター化されたコンストラクターを呼び出しています。
次のプログラムをコピーして、Subclass.java という名前のファイルに貼り付けます
例
ライブデモclass Superclass { int age; Superclass(int age) { this.age = age; } public void getAge() { System.out.println("The value of the variable named age in super class is: " +age); } } public class Subclass extends Superclass { Subclass(int age) { super(age); } public static void main(String args[]) { Subclass s = new Subclass(24); s.getAge(); } }
次の構文を使用して、上記のコードをコンパイルして実行します。
javac Subclass java Subclass
プログラムを実行すると、次の結果が得られます-
出力
The value of the variable named age in super class is: 24
IS-A 関係
IS-A は言い方です:このオブジェクトはそのオブジェクトの型です。 拡張がどのように行われるか見てみましょう キーワードは継承を実現するために使用されます。
public class Animal { } public class Mammal extends Animal { } public class Reptile extends Animal { } public class Dog extends Mammal { }
さて、上記の例に基づいて、オブジェクト指向の用語では、次のことが当てはまります-
- Animal は Mammal クラスのスーパークラスです。
- Animal は Reptile クラスのスーパークラスです。
- 哺乳類と爬虫類は動物クラスのサブクラスです。
- 犬は哺乳類クラスと動物クラスのサブクラスです。
ここで、IS-A 関係を考えると、次のように言えます −
- 哺乳類は動物です
- 爬虫類は動物です
- 犬は哺乳類です
- したがって:犬も動物です
extends キーワードを使用すると、サブクラスは、スーパークラスのプライベート プロパティを除く、スーパークラスのすべてのプロパティを継承できます。
インスタンス演算子を使用することで、哺乳動物が実際に動物であることを保証できます。
例
ライブデモclass Animal { } class Mammal extends Animal { } class Reptile extends Animal { } public class Dog extends Mammal { public static void main(String args[]) { Animal a = new Animal(); Mammal m = new Mammal(); Dog d = new Dog(); System.out.println(m instanceof Animal); System.out.println(d instanceof Mammal); System.out.println(d instanceof Animal); } }
これにより、次の結果が生成されます-
出力
true true true
extends についてよく理解しているため、 キーワード、実装の方法を見てみましょう キーワードは、IS-A 関係を取得するために使用されます。
一般的に、実装 キーワードは、インターフェイスのプロパティを継承するためにクラスで使用されます。インターフェイスはクラスによって拡張できません。
例
public interface Animal { } public class Mammal implements Animal { } public class Dog extends Mammal { }
キーワードのインスタンス
instanceof を使用しましょう Mammal が実際に Animal であり、dog が実際に Animal であるかどうかを判断する演算子。
例
ライブデモinterface Animal{} class Mammal implements Animal{} public class Dog extends Mammal { public static void main(String args[]) { Mammal m = new Mammal(); Dog d = new Dog(); System.out.println(m instanceof Animal); System.out.println(d instanceof Mammal); System.out.println(d instanceof Animal); } }
これにより、次の結果が生成されます-
出力
true true true
HAS-A 関係
これらの関係は、主に使用法に基づいています。これは、特定のクラスが HAS-A かどうかを決定します 特定のこと。この関係は、コードの重複とバグを減らすのに役立ちます。
例を見てみましょう −
例
public class Vehicle{} public class Speed{} public class Van extends Vehicle { private Speed sp; }
これは、クラス Van HAS-A Speed であることを示しています。 Speed 用に別のクラスを用意することで、Speed に属するコード全体を Van クラス内に配置する必要がなくなり、Speed クラスを複数のアプリケーションで再利用できるようになります。
オブジェクト指向機能では、ユーザーはどのオブジェクトが実際の作業を行っているかを気にする必要はありません。これを実現するために、Van クラスは実装の詳細を Van クラスのユーザーから隠します。したがって、基本的には、ユーザーが Van クラスに特定のアクションを実行するように依頼すると、Van クラスは自分で作業を行うか、別のクラスにアクションの実行を依頼します。
継承の種類
以下に示すように、さまざまな種類の継承があります。
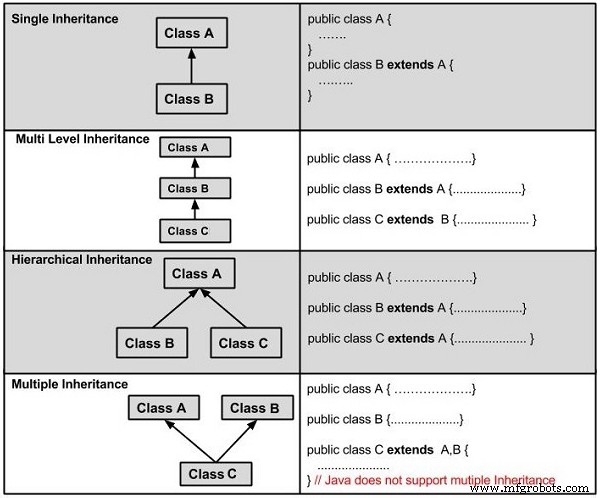
覚えておくべき非常に重要な事実は、Java が多重継承をサポートしていないということです。これは、クラスが複数のクラスを拡張できないことを意味します。したがって、以下は違法です −
例
public class extends Animal, Mammal{}
ただし、クラスは 1 つ以上のインターフェースを実装できるため、Java は多重継承の不可能性を取り除くことができました。
Java