Python タプル
Python タプル
この記事では、Python タプルに関するすべてを学びます。より具体的には、タプルとは何か、タプルを作成する方法、タプルをいつ使用するか、および知っておくべきさまざまな方法について説明します。
ビデオ:Python のリストとタプル
Python のタプルはリストに似ています。この 2 つの違いは、一度割り当てられたタプルの要素は変更できないのに対し、リストの要素は変更できることです。
タプルの作成
タプルは、すべての項目 (要素) を括弧 ()
内に配置することによって作成されます 、 カンマで区切られた。括弧はオプションですが、使用することをお勧めします。
タプルには任意の数の項目を含めることができ、さまざまな型 (整数、浮動小数点数、リスト、文字列など) にすることができます。
# Different types of tuples
# Empty tuple
my_tuple = ()
print(my_tuple)
# Tuple having integers
my_tuple = (1, 2, 3)
print(my_tuple)
# tuple with mixed datatypes
my_tuple = (1, "Hello", 3.4)
print(my_tuple)
# nested tuple
my_tuple = ("mouse", [8, 4, 6], (1, 2, 3))
print(my_tuple)
出力
() (1, 2, 3) (1, 'Hello', 3.4) ('mouse', [8, 4, 6], (1, 2, 3))
括弧を使用せずにタプルを作成することもできます。これはタプル パッキングと呼ばれます。
my_tuple = 3, 4.6, "dog"
print(my_tuple)
# tuple unpacking is also possible
a, b, c = my_tuple
print(a) # 3
print(b) # 4.6
print(c) # dog
出力
(3, 4.6, 'dog') 3 4.6 dog
要素が 1 つのタプルを作成するのは少し難しいです。
括弧内に 1 つの要素を含めるだけでは十分ではありません。実際にタプルであることを示すために、末尾のコンマが必要です。
my_tuple = ("hello")
print(type(my_tuple)) # <class 'str'>
# Creating a tuple having one element
my_tuple = ("hello",)
print(type(my_tuple)) # <class 'tuple'>
# Parentheses is optional
my_tuple = "hello",
print(type(my_tuple)) # <class 'tuple'>
出力
<class 'str'> <class 'tuple'> <class 'tuple'>
タプル要素へのアクセス
タプルの要素にアクセスするにはさまざまな方法があります。
1.索引付け
インデックス演算子 []
を使用できます インデックスが 0 から始まるタプル内の項目にアクセスします。
したがって、6 つの要素を持つタプルは 0 から 5 までのインデックスを持ちます。タプル インデックスの範囲外 (この例では 6,7,...) のインデックスにアクセスしようとすると、IndexError
が発生します。 .
インデックスは整数でなければならないため、float やその他の型は使用できません。これは TypeError
になります .
同様に、ネストされたタプルは、以下の例に示すように、ネストされたインデックスを使用してアクセスされます。
# Accessing tuple elements using indexing
my_tuple = ('p','e','r','m','i','t')
print(my_tuple[0]) # 'p'
print(my_tuple[5]) # 't'
# IndexError: list index out of range
# print(my_tuple[6])
# Index must be an integer
# TypeError: list indices must be integers, not float
# my_tuple[2.0]
# nested tuple
n_tuple = ("mouse", [8, 4, 6], (1, 2, 3))
# nested index
print(n_tuple[0][3]) # 's'
print(n_tuple[1][1]) # 4
出力
p t s 4
2.ネガティブ インデックス
Python では、そのシーケンスに負のインデックスを付けることができます。
-1 のインデックスは最後のアイテムを参照し、-2 は最後から 2 番目のアイテムを参照します。
# Negative indexing for accessing tuple elements
my_tuple = ('p', 'e', 'r', 'm', 'i', 't')
# Output: 't'
print(my_tuple[-1])
# Output: 'p'
print(my_tuple[-6])
出力
t p
3.スライス
スライス演算子コロン :
を使用して、タプル内のアイテムの範囲にアクセスできます .
# Accessing tuple elements using slicing
my_tuple = ('p','r','o','g','r','a','m','i','z')
# elements 2nd to 4th
# Output: ('r', 'o', 'g')
print(my_tuple[1:4])
# elements beginning to 2nd
# Output: ('p', 'r')
print(my_tuple[:-7])
# elements 8th to end
# Output: ('i', 'z')
print(my_tuple[7:])
# elements beginning to end
# Output: ('p', 'r', 'o', 'g', 'r', 'a', 'm', 'i', 'z')
print(my_tuple[:])
出力
('r', 'o', 'g') ('p', 'r') ('i', 'z') ('p', 'r', 'o', 'g', 'r', 'a', 'm', 'i', 'z')
以下に示すように、インデックスが要素間にあると考えると、スライスを最もよく視覚化できます。したがって、範囲にアクセスする場合は、タプルからその部分をスライスするインデックスが必要です。
<図>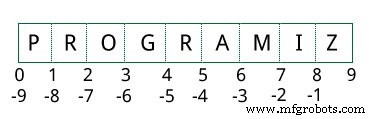
タプルの変更
リストとは異なり、タプルは不変です。
これは、一度割り当てられたタプルの要素を変更できないことを意味します。ただし、要素自体がリストのような変更可能なデータ型である場合は、ネストされた項目を変更できます。
タプルを異なる値に割り当てることもできます (再割り当て)。
# Changing tuple values
my_tuple = (4, 2, 3, [6, 5])
# TypeError: 'tuple' object does not support item assignment
# my_tuple[1] = 9
# However, item of mutable element can be changed
my_tuple[3][0] = 9 # Output: (4, 2, 3, [9, 5])
print(my_tuple)
# Tuples can be reassigned
my_tuple = ('p', 'r', 'o', 'g', 'r', 'a', 'm', 'i', 'z')
# Output: ('p', 'r', 'o', 'g', 'r', 'a', 'm', 'i', 'z')
print(my_tuple)
出力
(4, 2, 3, [9, 5]) ('p', 'r', 'o', 'g', 'r', 'a', 'm', 'i', 'z')
+
を使用できます 2 つのタプルを結合する演算子。これは連結と呼ばれます .
繰り返すこともできます *
を使用して、指定された回数のタプル内の要素
両方 +
と *
操作は新しいタプルになります。
# Concatenation
# Output: (1, 2, 3, 4, 5, 6)
print((1, 2, 3) + (4, 5, 6))
# Repeat
# Output: ('Repeat', 'Repeat', 'Repeat')
print(("Repeat",) * 3)
出力
(1, 2, 3, 4, 5, 6) ('Repeat', 'Repeat', 'Repeat')
タプルの削除
上で説明したように、タプルの要素を変更することはできません。これは、タプルからアイテムを削除または除去できないことを意味します。
ただし、キーワード del を使用してタプルを完全に削除することは可能です。
# Deleting tuples
my_tuple = ('p', 'r', 'o', 'g', 'r', 'a', 'm', 'i', 'z')
# can't delete items
# TypeError: 'tuple' object doesn't support item deletion
# del my_tuple[3]
# Can delete an entire tuple
del my_tuple
# NameError: name 'my_tuple' is not defined
print(my_tuple)
出力
Traceback (most recent call last): File "<string>", line 12, in <module> NameError: name 'my_tuple' is not defined
タプル メソッド
タプルでは、項目を追加または削除するメソッドは使用できません。次の 2 つの方法のみが利用可能です。
Python タプル メソッドの例:
my_tuple = ('a', 'p', 'p', 'l', 'e',)
print(my_tuple.count('p')) # Output: 2
print(my_tuple.index('l')) # Output: 3
出力
2 3
その他のタプル操作
1.タプル メンバーシップ テスト
キーワード in
を使用して、アイテムがタプルに存在するかどうかをテストできます .
# Membership test in tuple
my_tuple = ('a', 'p', 'p', 'l', 'e',)
# In operation
print('a' in my_tuple)
print('b' in my_tuple)
# Not in operation
print('g' not in my_tuple)
出力
True False True
2.タプルの繰り返し
for
を使用できます タプル内の各項目を反復処理するループ。
# Using a for loop to iterate through a tuple
for name in ('John', 'Kate'):
print("Hello", name)
出力
Hello John Hello Kate
リストよりもタプルの利点
タプルはリストと非常に似ているため、どちらも同じような状況で使用されます。ただし、リストよりもタプルを実装することにはいくつかの利点があります。主な利点の一部を以下に示します:
- 通常、異種 (異なる) データ型にはタプルを使用し、同種 (類似) データ型にはリストを使用します。
- タプルは不変であるため、タプルの繰り返しはリストよりも高速です。そのため、パフォーマンスがわずかに向上します。
- 不変要素を含むタプルは、辞書のキーとして使用できます。リストでは、これは不可能です。
- 変更されないデータがある場合、それをタプルとして実装すると、書き込み保護が維持されることが保証されます。
Python