パイソン セット
Python セット
このチュートリアルでは、Python セットに関するすべてを学びます。作成方法、要素の追加または削除、および Python でセットに対して実行されるすべての操作。
ビデオ:Python のセット
セットは、順序付けされていないアイテムのコレクションです。すべてのセット要素は一意 (重複なし) であり、不変 (変更不可) でなければなりません。
ただし、セット自体は変更可能です。項目を追加または削除できます。
集合は、和集合、交差、対称差などの数学的集合操作の実行にも使用できます。
Python セットの作成
セットは、すべての項目 (要素) を中括弧 {}
内に配置することによって作成されます 、コンマで区切るか、組み込みの set()
を使用して 関数。
任意の数の項目を持つことができ、それらは異なる型 (整数、浮動小数点数、タプル、文字列など) である可能性があります。ただし、セットには、リスト、セット、辞書などの可変要素を要素として含めることはできません。
# Different types of sets in Python
# set of integers
my_set = {1, 2, 3}
print(my_set)
# set of mixed datatypes
my_set = {1.0, "Hello", (1, 2, 3)}
print(my_set)
出力
{1, 2, 3} {1.0, (1, 2, 3), 'Hello'}
以下の例も試してみてください。
# set cannot have duplicates
# Output: {1, 2, 3, 4}
my_set = {1, 2, 3, 4, 3, 2}
print(my_set)
# we can make set from a list
# Output: {1, 2, 3}
my_set = set([1, 2, 3, 2])
print(my_set)
# set cannot have mutable items
# here [3, 4] is a mutable list
# this will cause an error.
my_set = {1, 2, [3, 4]}
出力
{1, 2, 3, 4} {1, 2, 3} Traceback (most recent call last): File "<string>", line 15, in <module> my_set = {1, 2, [3, 4]} TypeError: unhashable type: 'list'
空のセットを作成するのは少し難しいです。
空の中括弧 {}
Python で空の辞書を作成します。要素なしでセットを作成するには、 set()
を使用します 引数なしの関数。
# Distinguish set and dictionary while creating empty set
# initialize a with {}
a = {}
# check data type of a
print(type(a))
# initialize a with set()
a = set()
# check data type of a
print(type(a))
出力
<class 'dict'> <class 'set'>
Python でのセットの変更
セットは可変です。ただし、それらは順序付けされていないため、インデックスを作成しても意味がありません。
インデックス付けやスライスを使用してセットの要素にアクセスしたり変更したりすることはできません。セットデータ型はそれをサポートしていません.
add()
を使用して単一の要素を追加できます メソッド、および update()
を使用した複数の要素 方法。 update()
メソッドは、タプル、リスト、文字列、またはその他のセットを引数として取ることができます。いずれの場合も、重複は回避されます。
# initialize my_set
my_set = {1, 3}
print(my_set)
# my_set[0]
# if you uncomment the above line
# you will get an error
# TypeError: 'set' object does not support indexing
# add an element
# Output: {1, 2, 3}
my_set.add(2)
print(my_set)
# add multiple elements
# Output: {1, 2, 3, 4}
my_set.update([2, 3, 4])
print(my_set)
# add list and set
# Output: {1, 2, 3, 4, 5, 6, 8}
my_set.update([4, 5], {1, 6, 8})
print(my_set)
出力
{1, 3} {1, 2, 3} {1, 2, 3, 4} {1, 2, 3, 4, 5, 6, 8}
セットから要素を削除する
メソッド discard()
を使用して、特定のアイテムをセットから削除できます。 と remove()
.
2 つの唯一の違いは、discard()
要素がセットに存在しない場合、関数はセットを変更しないままにします。一方、remove()
関数はそのような状態でエラーを発生させます (要素がセットに存在しない場合)。
次の例はこれを示しています。
# Difference between discard() and remove()
# initialize my_set
my_set = {1, 3, 4, 5, 6}
print(my_set)
# discard an element
# Output: {1, 3, 5, 6}
my_set.discard(4)
print(my_set)
# remove an element
# Output: {1, 3, 5}
my_set.remove(6)
print(my_set)
# discard an element
# not present in my_set
# Output: {1, 3, 5}
my_set.discard(2)
print(my_set)
# remove an element
# not present in my_set
# you will get an error.
# Output: KeyError
my_set.remove(2)
出力
{1, 3, 4, 5, 6} {1, 3, 5, 6} {1, 3, 5} {1, 3, 5} Traceback (most recent call last): File "<string>", line 28, in <module> KeyError: 2
同様に、 pop()
を使用してアイテムを削除して返すことができます メソッド。
set は順不同のデータ型であるため、どのアイテムがポップされるかを決定する方法はありません。それは完全に任意です。
clear()
を使用して、セットからすべてのアイテムを削除することもできます メソッド。
# initialize my_set
# Output: set of unique elements
my_set = set("HelloWorld")
print(my_set)
# pop an element
# Output: random element
print(my_set.pop())
# pop another element
my_set.pop()
print(my_set)
# clear my_set
# Output: set()
my_set.clear()
print(my_set)
print(my_set)
出力
{'H', 'l', 'r', 'W', 'o', 'd', 'e'} H {'r', 'W', 'o', 'd', 'e'} set()
Python セット オペレーション
集合は、和集合、交差、差、対称差などの数学的集合操作を実行するために使用できます。演算子またはメソッドでこれを行うことができます。
次の操作について、次の 2 つのセットを考えてみましょう。
>>> A = {1, 2, 3, 4, 5}
>>> B = {4, 5, 6, 7, 8}
ユニオンを設定
<図>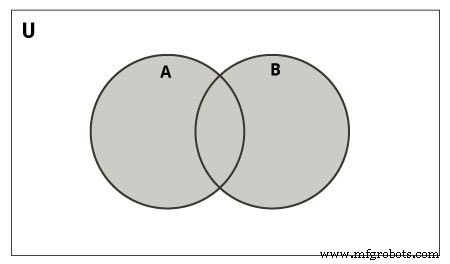
Aの連合 と B 両方のセットからのすべての要素のセットです。
ユニオンは |
を使用して実行されます オペレーター。 union()
を使用して同じことが実現できます メソッド。
# Set union method
# initialize A and B
A = {1, 2, 3, 4, 5}
B = {4, 5, 6, 7, 8}
# use | operator
# Output: {1, 2, 3, 4, 5, 6, 7, 8}
print(A | B)
出力
{1, 2, 3, 4, 5, 6, 7, 8}
Python シェルで次の例を試してください。
# use union function
>>> A.union(B)
{1, 2, 3, 4, 5, 6, 7, 8}
# use union function on B
>>> B.union(A)
{1, 2, 3, 4, 5, 6, 7, 8}
交差点を設定
<図>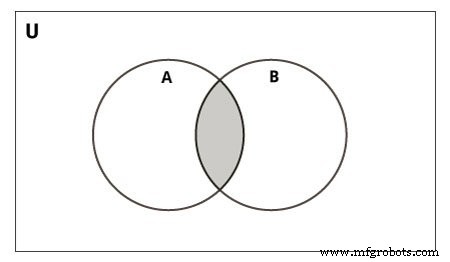
A の交差点 と B 両方のセットに共通する要素のセットです。
交差は &
を使用して実行されます オペレーター。 intersection()
を使用して同じことが実現できます メソッド。
# Intersection of sets
# initialize A and B
A = {1, 2, 3, 4, 5}
B = {4, 5, 6, 7, 8}
# use & operator
# Output: {4, 5}
print(A & B)
出力
{4, 5}
Python シェルで次の例を試してください。
# use intersection function on A
>>> A.intersection(B)
{4, 5}
# use intersection function on B
>>> B.intersection(A)
{4, 5}
設定差
<図>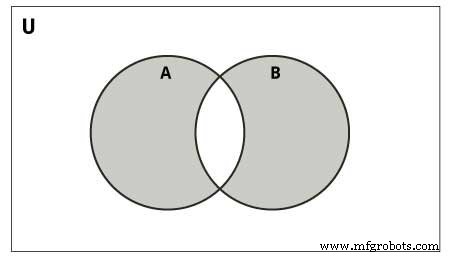
セット B の違い セット A から (A - B ) は A のみにある要素のセットです しかし、B にはありません .同様に、B - A B の要素のセットです しかし、A にはありません .
差分は -
を使用して実行されます オペレーター。 difference()
を使用して同じことが実現できます メソッド。
# Difference of two sets
# initialize A and B
A = {1, 2, 3, 4, 5}
B = {4, 5, 6, 7, 8}
# use - operator on A
# Output: {1, 2, 3}
print(A - B)
出力
{1, 2, 3}
Python シェルで次の例を試してください。
# use difference function on A
>>> A.difference(B)
{1, 2, 3}
# use - operator on B
>>> B - A
{8, 6, 7}
# use difference function on B
>>> B.difference(A)
{8, 6, 7}
対称差を設定
<図>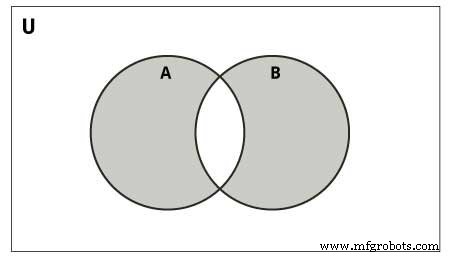
A の対称差 と B A の要素のセットです と B ただし、両方ではありません (交差点を除く)。
^
を使用して対称差分を実行します オペレーター。メソッド symmetric_difference()
を使用して同じことが実現できます .
# Symmetric difference of two sets
# initialize A and B
A = {1, 2, 3, 4, 5}
B = {4, 5, 6, 7, 8}
# use ^ operator
# Output: {1, 2, 3, 6, 7, 8}
print(A ^ B)
出力
{1, 2, 3, 6, 7, 8}
Python シェルで次の例を試してください。
# use symmetric_difference function on A
>>> A.symmetric_difference(B)
{1, 2, 3, 6, 7, 8}
# use symmetric_difference function on B
>>> B.symmetric_difference(A)
{1, 2, 3, 6, 7, 8}
その他の Python セット メソッド
多くの set メソッドがあり、そのうちのいくつかは既に上で使用しています。以下は、セット オブジェクトで使用できるすべてのメソッドのリストです:
メソッド | 説明 |
---|---|
add() | 要素をセットに追加します |
clear() | セットからすべての要素を削除します |
copy() | セットのコピーを返します |
difference() | 2 つ以上のセットの差を新しいセットとして返します |
difference_update() | このセットから別のセットのすべての要素を削除します |
discard() | 要素がメンバーである場合、要素をセットから削除します。 (要素が設定されていない場合は何もしません) |
交差点() | 2 つのセットの共通部分を新しいセットとして返します |
intersection_update() | それ自体と別の交差でセットを更新します |
isdisjoint() | True を返します 2 つのセットの交差が null の場合 |
issubset() | True を返します 別のセットにこのセットが含まれている場合 |
issuperset() | True を返します このセットに別のセットが含まれている場合 |
pop() | 任意のセット要素を削除して返します。 KeyError を上げる セットが空の場合 |
remove() | セットから要素を削除します。要素がメンバーでない場合は、KeyError が発生します |
symmetric_difference() | 2 つのセットの対称差を新しいセットとして返します |
symmetric_difference_update() | それ自体と別の対称差でセットを更新します |
union() | セットの結合を新しいセットで返します |
update() | それ自体と他のものを結合してセットを更新します |
その他のセット操作
メンバーシップ テストを設定
in
を使用して、アイテムがセットに存在するかどうかをテストできます キーワード。
# in keyword in a set
# initialize my_set
my_set = set("apple")
# check if 'a' is present
# Output: True
print('a' in my_set)
# check if 'p' is present
# Output: False
print('p' not in my_set)
出力
True False
セットの繰り返し
for
を使用して、セット内の各アイテムを反復処理できます ループ。
>>> for letter in set("apple"):
... print(letter)
...
a
p
e
l
Set を使用した組み込み関数
all()
などの組み込み関数 、 any()
、 enumerate()
、 len()
、 max()
、 min()
、 sorted()
、 sum()
などは、さまざまなタスクを実行するためにセットで一般的に使用されます。
関数 | 説明 |
---|---|
all() | True を返します セットのすべての要素が true の場合 (またはセットが空の場合)。 |
any() | True を返します セットのいずれかの要素が true の場合。セットが空の場合、False を返します . |
enumerate() | 列挙オブジェクトを返します。セットのすべてのアイテムのインデックスと値がペアとして含まれています。 |
len() | セット内の長さ (アイテムの数) を返します。 |
max() | セット内の最大のアイテムを返します。 |
min() | セット内の最小のアイテムを返します。 |
sorted() | セット内の要素から新しいソート済みリストを返します (セット自体はソートしません)。 |
sum() | セット内のすべての要素の合計を返します。 |
Python 冷凍セット
Frozenset は、セットの特性を持つ新しいクラスですが、その要素は一度割り当てられると変更できません。タプルは不変のリストですが、frozenset は不変のセットです。
変更可能なセットはハッシュ化できないため、辞書のキーとして使用できません。一方、frozenset はハッシュ可能で、辞書のキーとして使用できます。
凍結セットは、frozenset() 関数を使用して作成できます。
このデータ型は copy()
のようなメソッドをサポートしています 、 difference()
、 intersection()
、 isdisjoint()
、 issubset()
、 issuperset()
、 symmetric_difference()
と union()
.不変であるため、要素を追加または削除するメソッドはありません。
# Frozensets
# initialize A and B
A = frozenset([1, 2, 3, 4])
B = frozenset([3, 4, 5, 6])
これらの例を Python シェルで試してください。
>>> A.isdisjoint(B)
False
>>> A.difference(B)
frozenset({1, 2})
>>> A | B
frozenset({1, 2, 3, 4, 5, 6})
>>> A.add(3)
...
AttributeError: 'frozenset' object has no attribute 'add'
Python