C++ 文字列
C++ は、次の 2 種類の文字列表現を提供します −
- C スタイルの文字列
- 標準 C++ で導入された文字列クラス タイプ。
C スタイルの文字列
C スタイルの文字列は C 言語で生まれ、C++ でも引き続きサポートされています。この文字列は、実際には null で終わる文字の 1 次元配列です。 文字 '\0'。したがって、null で終わる文字列には、文字列を構成する文字とそれに続く null が含まれます。 .
次の宣言と初期化により、「Hello」という単語からなる文字列が作成されます。配列の最後にヌル文字を保持するために、文字列を含む文字配列のサイズは、単語「Hello」の文字数よりも 1 大きくなります。
char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
配列の初期化の規則に従えば、上記のステートメントを次のように書くことができます −
char greeting[] = "Hello";
以下は、上記で定義された文字列の C/C++ でのメモリ表示です −
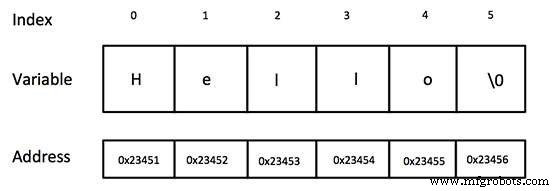
実際には、文字列定数の末尾にヌル文字を配置しません。 C++ コンパイラは、配列を初期化するときに、文字列の末尾に '\0' を自動的に配置します。上記の文字列を印刷してみましょう −
ライブデモ#include <iostream> using namespace std; int main () { char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'}; cout << "Greeting message: "; cout << greeting << endl; return 0; }
上記のコードをコンパイルして実行すると、次の結果が生成されます −
Greeting message: Hello
C++ は、ヌル終了文字列を操作する幅広い関数をサポートしています −
Sr.No | 機能と目的 |
---|---|
1 | strcpy(s1, s2); 文字列 s2 を文字列 s1 にコピーします。 |
2 | strcat(s1, s2); 文字列 s2 を文字列 s1 の末尾に連結します。 |
3 | strlen(s1); 文字列 s1 の長さを返します。 |
4 | strcmp(s1, s2);
s1 と s2 が同じ場合は 0 を返します。 s1 |
5 | strchr(s1, ch); 文字列 s1 で文字 ch が最初に出現する位置へのポインターを返します。 |
6 | strstr(s1, s2); 文字列 s1 で最初に出現する文字列 s2 へのポインターを返します。 |
次の例は、上記の関数のいくつかを利用しています-
ライブデモ#include <iostream> #include <cstring> using namespace std; int main () { char str1[10] = "Hello"; char str2[10] = "World"; char str3[10]; int len ; // copy str1 into str3 strcpy( str3, str1); cout << "strcpy( str3, str1) : " << str3 << endl; // concatenates str1 and str2 strcat( str1, str2); cout << "strcat( str1, str2): " << str1 << endl; // total lenghth of str1 after concatenation len = strlen(str1); cout << "strlen(str1) : " << len << endl; return 0; }
上記のコードをコンパイルして実行すると、次のような結果が生成されます −
strcpy( str3, str1) : Hello strcat( str1, str2): HelloWorld strlen(str1) : 10
C++ の文字列クラス
標準 C++ ライブラリは 文字列 を提供します 上記のすべての操作をサポートし、さらに多くの機能をサポートするクラス タイプ。次の例を確認してみましょう-
ライブデモ#include <iostream> #include <string> using namespace std; int main () { string str1 = "Hello"; string str2 = "World"; string str3; int len ; // copy str1 into str3 str3 = str1; cout << "str3 : " << str3 << endl; // concatenates str1 and str2 str3 = str1 + str2; cout << "str1 + str2 : " << str3 << endl; // total length of str3 after concatenation len = str3.size(); cout << "str3.size() : " << len << endl; return 0; }
上記のコードをコンパイルして実行すると、次のような結果が生成されます −
str3 : Hello str1 + str2 : HelloWorld str3.size() : 10
C言語